MoveIt2 Interface and API

Overview
This package contains a small API modeled after the Move Group C++ Interface Tutorial that allows a user to command desired end-effector poses to an Interbotix arm. It is not meant to be all-encompassing but rather should be viewed as a starting point for someone interested in creating their own MoveIt interface to interact with an arm. The package also contains a small GUI that can be used to pose the end-effector.
Finally, this package also contains a modified version of the Move Group Python Interface Tutorial script that can be used as a guide for those users who would like to interface with an Interbotix robot via the MoveIt Commander Python module.
Note
Only the C++ interface has been ported to ROS 2. The Python interface is waiting on MoveIt Commander to be ported.
Structure
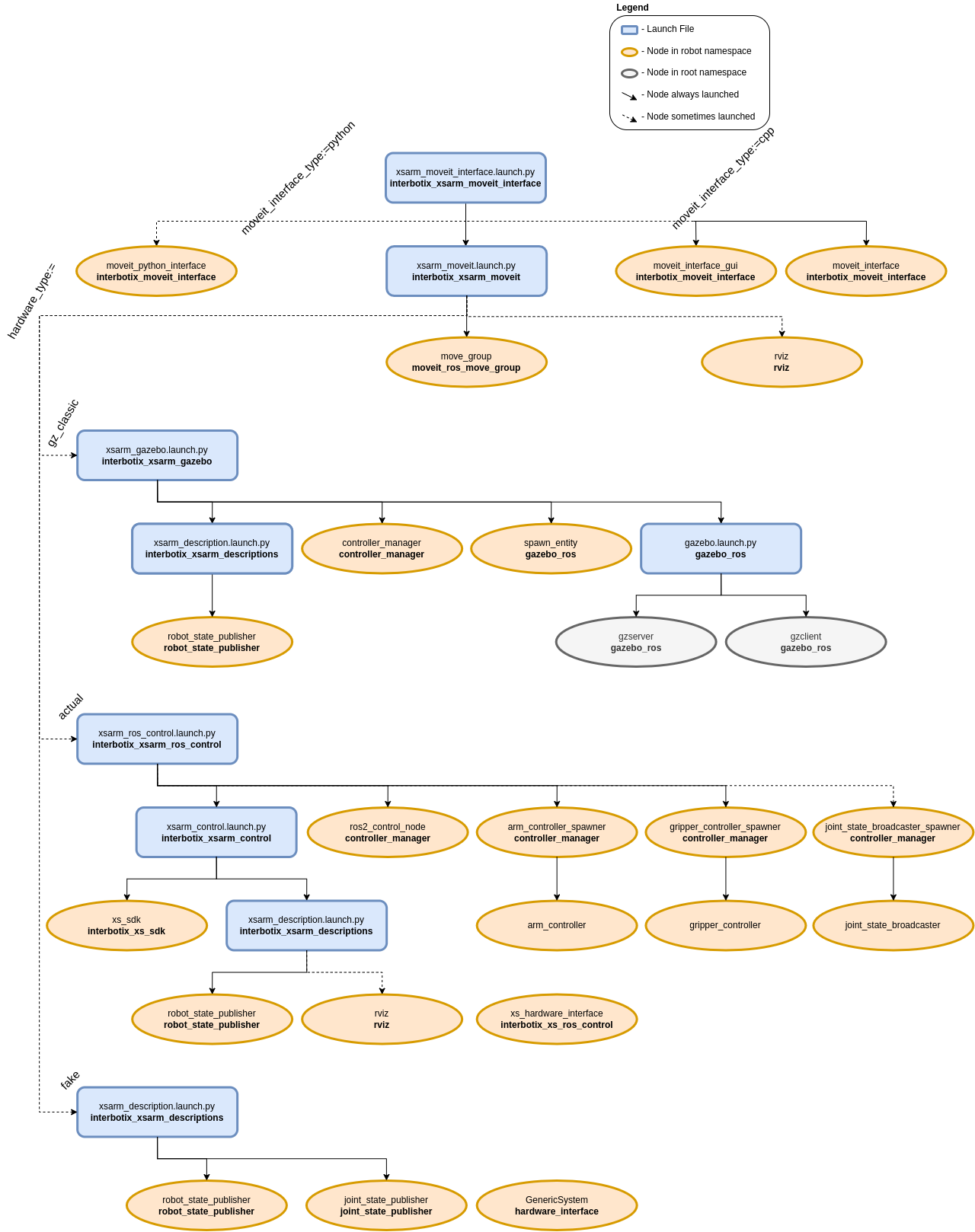
As shown above, this package builds on top of of the interbotix_xsarm_moveit package. To get familiar with that package, please refer to its documentation. The interbotix_xsarm_moveit_interface nodes are described below:
- moveit_interface - a small C++ API that makes it easier for a user to command custom poses to the end-effector of an Interbotix arm; it uses MoveIt’s planner behind the scenes to generate desired joint trajectories
- moveit_interface_gui - a GUI (modeled after the one in the joint_state_publisher_gui package) that allows a user to enter in desired end-effector poses via text fields or sliders; it uses the moveit_interface API to plan and execute trajectories
- moveit_python_interface - a modified version of the script used in the Move Group Python Interface Tutorial that is meant to work with an Interbotix arm; just press ‘Enter’ in the terminal to walk through the different steps; the desired joint state goal, pose goal, and end-effector offset (when attaching the box) are stored in YAML files located in the config directory
Note
Note that all the nodes defined above live in the interbotix_ros_toolboxes repo. That is because these nodes, besides for being used here, are also used in other repositories (like interbotix_ros_rovers). To make it easier to maintain, it made more sense to keep one instance of them in the toolbox repository instead of having duplicates in multiple repositories.
Usage
To run this package on the physical robot, type the line below in a terminal (assuming the ViperX-300 is being launched).
$ ros2 launch interbotix_xsarm_moveit_interface xsarm_moveit_interface.launch.py robot_model:=vx300
A GUI should pop-up similar to the one below. In it, a user should specify the desired position and orientation of the end-effector (as defined by the ‘ee_gripper_link’ w.r.t. the ‘world’ frame). This can be done either via the slider bars or by entering values into the text fields. Next, a user can press one of five buttons. They are:
- Plan Pose - MoveIt will attempt to find a trajectory that places the end-effector at the desired position and orientation
- Plan Position - MoveIt will attempt to find a trajectory that places the end-effector at the desired position, ignoring orientation
- Plan Orientation - MoveIt will attempt to find a trajectory that places the end-effector at the desired orientation, ignoring position
- Execute - Once a valid plan has been found, this button can be pressed to actually execute the trajectory on the robot
- Reset - This resets all slider bars to the middle and text fields to ‘0.00’
- Clear Markers - This resets all MoveIt visual tools displays
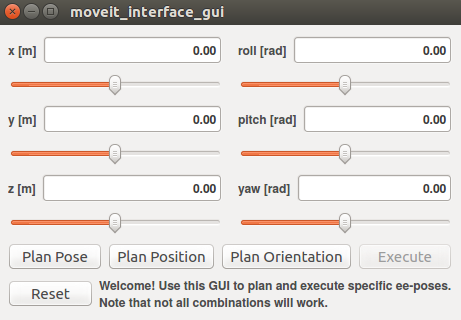
Note
Occasionally, MoveIt will be unsuccessful in finding a valid trajectory. This could be for one of two reasons. Either the desired pose is not physically attainable by the arm or the random seed generated by MoveIt was a bad guess. If it is clear that the pose is physically attainable, try hitting the desired ‘Plan’ button a few more times.
Note
It is significantly more difficult for the planner to find a valid trajectory when specifying poses and orientations rather than just positions, especially for lower dof arms.
This is the bare minimum needed to get up and running. Take a look at the table below to see how to further customize with other launch file arguments.
Argument | Description | Default | Choices |
---|---|---|---|
robot_model | model type of the Interbotix Arm such as ‘wx200’ or ‘rx150’. | px100 , px150 , rx150 , rx200 , wx200 , wx250 , wx250s , vx250 , vx300 , vx300s , mobile_px100 , mobile_wx200 , mobile_wx250s |
|
robot_name | name of the robot (typically equal to robot_model , but could be anything). |
LaunchConfig(robot_model ) |
|
external_srdf_loc | the file path to the custom semantic description file that you would like to include in the Interbotix robot’s semantic description. | ‘’ | |
mode_configs | the file path to the ‘mode config’ YAML file. | LocalVar(‘FindPackageShare(pkg= interbotix_xsarm_moveit_interface ) + ‘config’ + ‘modes.yaml’’) |
|
use_moveit_rviz | launches RViz with MoveIt’s RViz configuration. | true |
true , false |
rviz_frame | defines the fixed frame parameter in RViz. Note that if use_world_frame is false , this parameter should be changed to a frame that exists. |
world |
|
rviz_config_file | file path to the config file RViz should load. | LocalVar(‘FindPackageShare(pkg= interbotix_xsarm_moveit_interface ) + ‘rviz’ + ‘xsarm_moveit_interface.rviz’’) |
|
world_filepath | the file path to the Gazebo ‘world’ file to load. | LocalVar(‘FindPackageShare(pkg= interbotix_common_sim ) + ‘worlds’ + ‘interbotix.world’’) |
|
moveit_interface_type | if ‘cpp’, launches the custom moveit_interface C++ API node; if ‘python’, launch the Python Interface tutorial node; only the cpp option is currently supported. | cpp |
cpp , |
use_moveit_interface_gui | launch a custom GUI to interface with the moveit_interface node so that the user can command specific end-effector poses (defined by ‘ee_gripper_link’). | true |
true , false |
use_sim_time | tells ROS nodes asking for time to get the Gazebo-published simulation time, published over the ROS topic /clock; this value is automatically set to ‘true’ if using Gazebo hardware. | false |
true , false |
base_link_frame | name of the ‘root’ link on the arm; typically base_link , but can be changed if attaching the arm to a mobile base that already has a base_link frame. |
base_link |
|
use_gripper | if true , the default gripper is included in the robot_description parameter; if false , it is left out; set to false if not using the default gripper. |
true |
true , false |
show_ar_tag | if true , the AR tag mount is included in the robot_description parameter; if false , it is left out; set to true if using the AR tag mount in your project. |
false |
true , false |
show_gripper_bar | if true , the gripper_bar link is included in the robot_description parameter; if false , the gripper_bar and finger links are not loaded. Set to false if you have a custom gripper attachment. |
true |
true , false |
show_gripper_fingers | if true, the gripper fingers are included in the robot_description parameter; if false , the gripper finger links are not loaded. Set to false if you have custom gripper fingers. |
true |
true , false |
use_world_frame | set this to true if you would like to load a ‘world’ frame to the robot_description parameter which is located exactly at the ‘base_link’ frame of the robot; if using multiple robots or if you would like to attach the ‘base_link’ frame of the robot to a different frame, set this to false . |
true |
true , false |
external_urdf_loc | the file path to the custom urdf.xacro file that you would like to include in the Interbotix robot’s urdf.xacro file. | ‘’ | |
hardware_type | configures the robot_description parameter to use the actual hardware, fake hardware, or hardware simulated in Gazebo. |
actual |
actual , fake , gz_classic |
robot_description | URDF of the robot; this is typically generated by the xacro command. | Command(FindExec(xacro ) + ‘ ‘ + LocalVar(‘FindPackageShare(pkg= interbotix_xsarm_descriptions ) + ‘urdf’ + LaunchConfig(robot_model )’) + ‘.urdf.xacro ‘ + ‘robot_name:=’ + LaunchConfig(robot_name ) + ‘ ‘ + ‘base_link_frame:=’ + LaunchConfig(base_link_frame ) + ‘ ‘ + ‘use_gripper:=’ + LaunchConfig(use_gripper ) + ‘ ‘ + ‘show_ar_tag:=’ + LaunchConfig(show_ar_tag ) + ‘ ‘ + ‘show_gripper_bar:=’ + LaunchConfig(show_gripper_bar ) + ‘ ‘ + ‘show_gripper_fingers:=’ + LaunchConfig(show_gripper_fingers ) + ‘ ‘ + ‘use_world_frame:=’ + LaunchConfig(use_world_frame ) + ‘ ‘ + ‘external_urdf_loc:=’ + LaunchConfig(external_urdf_loc ) + ‘ ‘ + ‘hardware_type:=’ + LaunchConfig(hardware_type ) + ‘ ‘) |